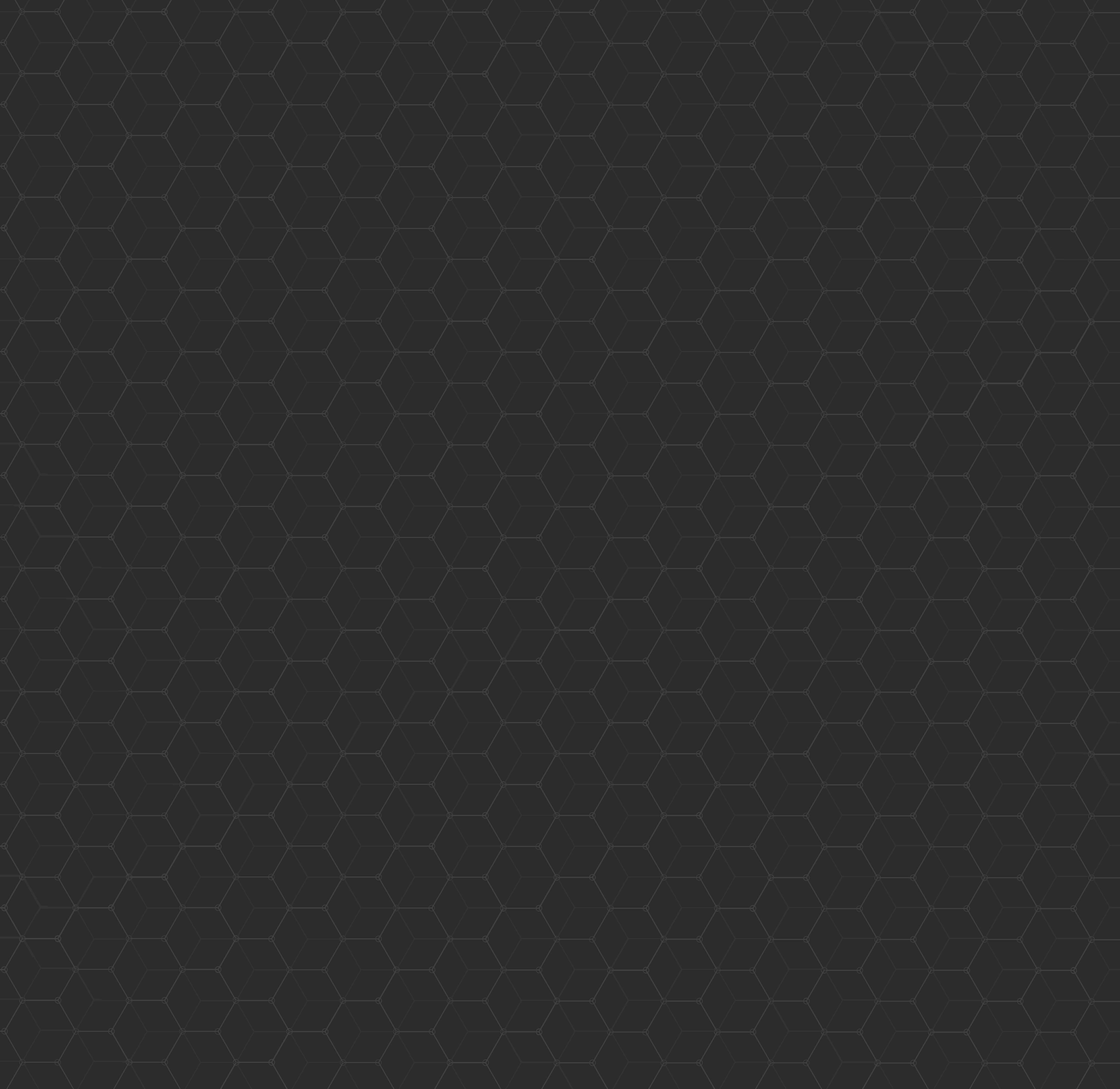
Self-Paced Course
Natural Language Processing in Python
Learn NLP in Python, including text preprocessing, machine learning, transformers & LLMs using scikit-learn, spaCy & Hugging Face
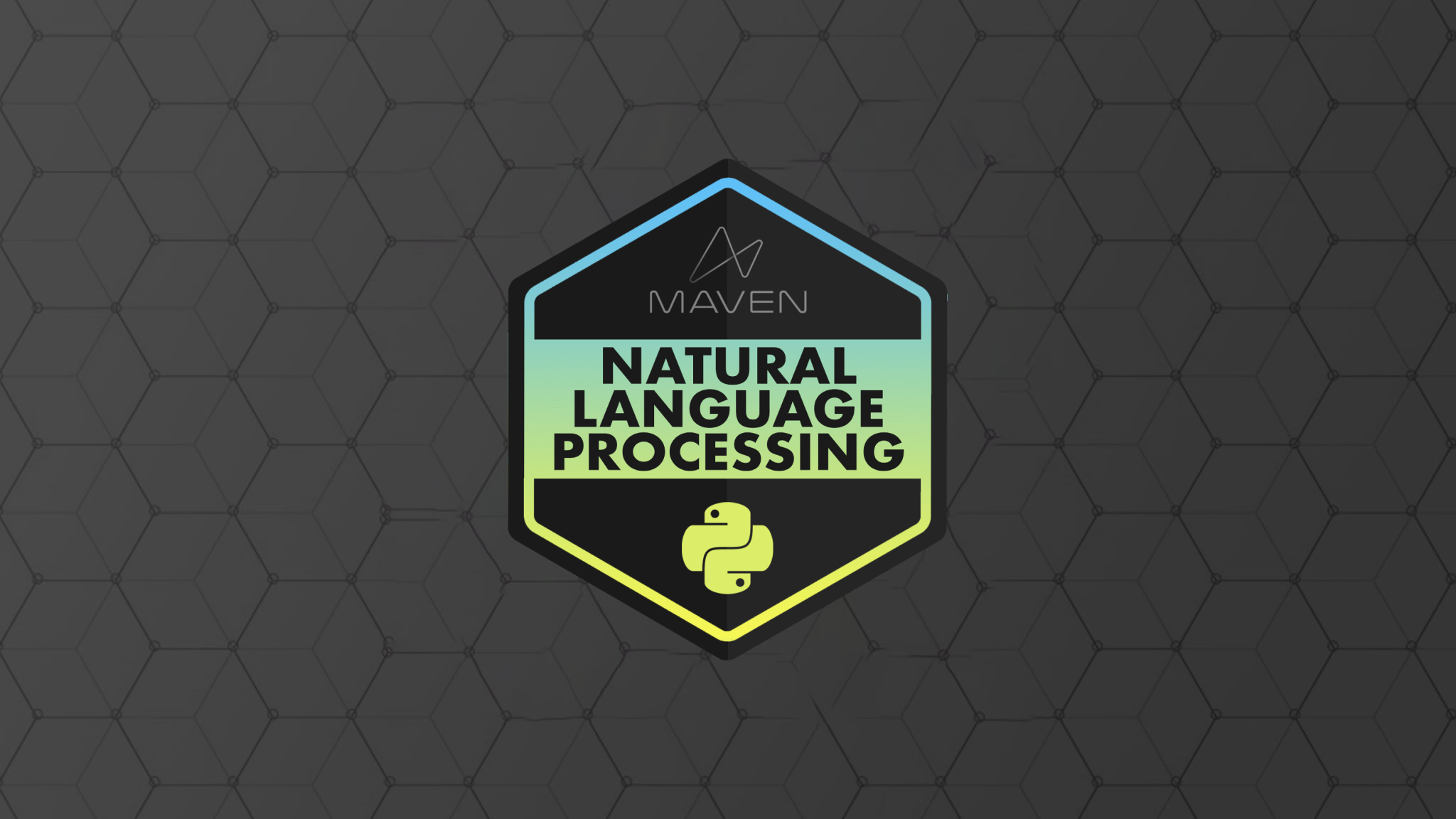
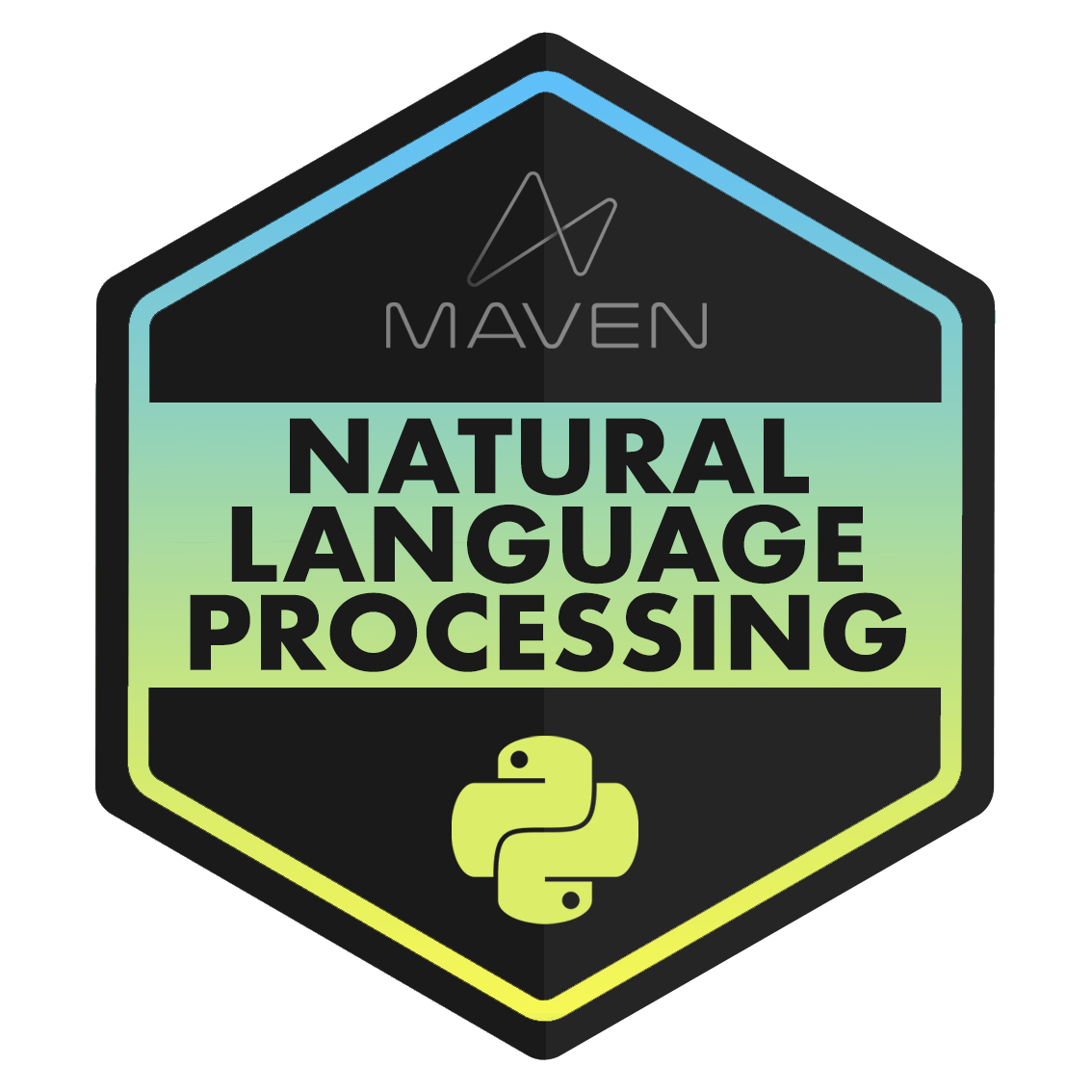
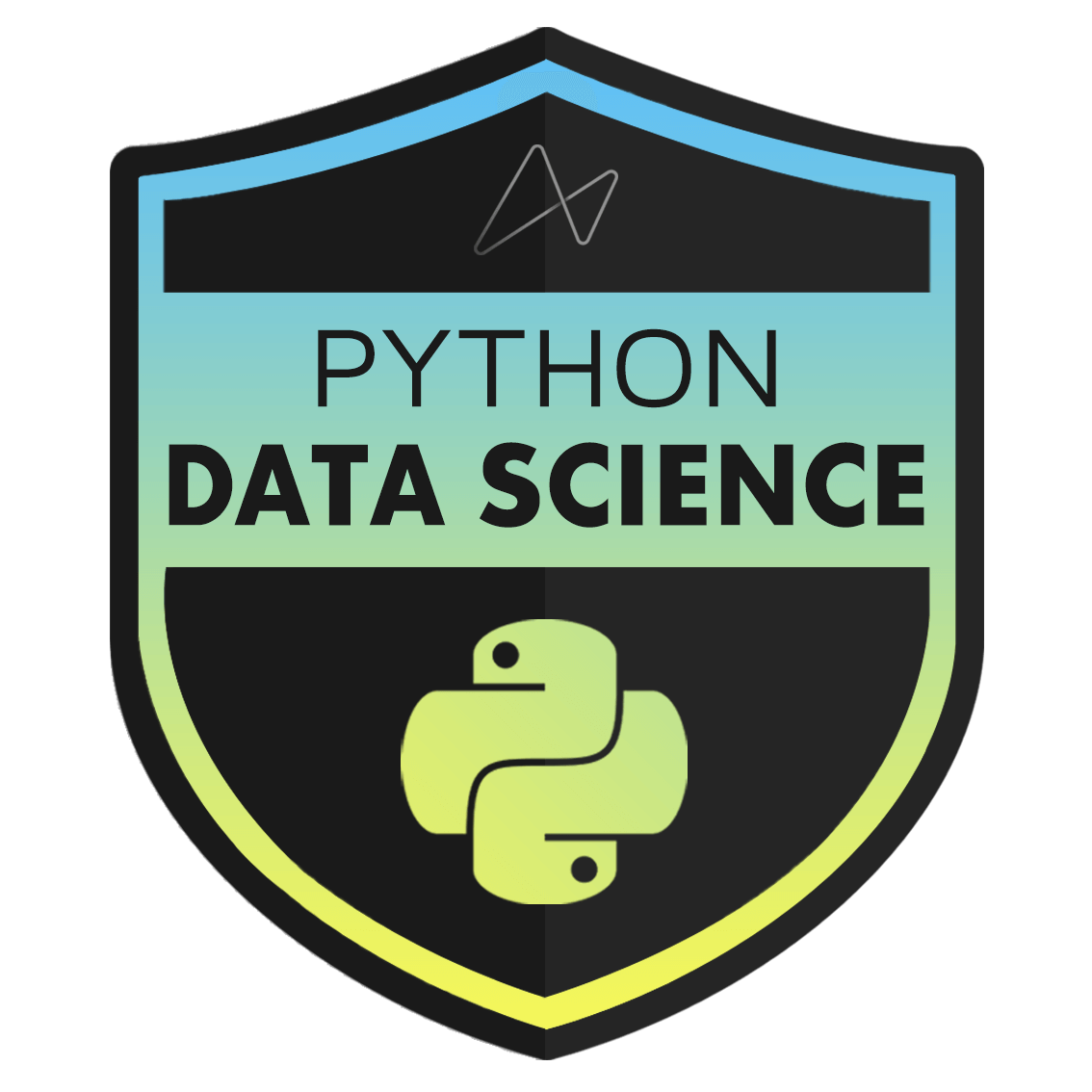
Course Description
This is a practical, hands-on course designed to give you a comprehensive overview of all the essential concepts for Natural Language Processing (NLP) in Python.
We’ll start by reviewing the history and evolution of NLP over the past 70 years, including the most popular architecture at the moment, Transformers. We'll also walk through the initial text preprocessing steps required for modeling, where you’ll learn how to clean and normalize data with pandas and spaCy, then vectorize that data into a Document-Term Matrix using both word counts and TF-IDF scores.
From there, the course is split into two parts: the first half covers traditional machine learning techniques and the second half covers modern deep learning and LLM (large language model) approaches.
For the traditional NLP applications, we'll begin with Sentiment Analysis to determine the positivity or negativity of text using the VADER library. Then we’ll cover Text Classification on labeled data with Naïve Bayes, as well as Topic Modeling on unlabeled data using Non-Negative Matrix Factorization, all using the scikit-learn library.
Once you have a solid understanding of the foundational NLP concepts, we’ll move on to the second half of the course on modern NLP techniques, which covers the major advancements in NLP and the data science mindset shift over the past decade.
We’ll start with the basic building blocks of modern NLP techniques, which are neural networks. You’ll learn how neural networks are trained, become familiar with key terms like layers, nodes, weights, and activation functions, and then get introduced to popular deep learning architectures and their practical applications.
From there, we’ll talk about Transformers, the architectures behind popular LLMs like ChatGPT, Gemini, and Claude. We’ll cover how the main layers work and what they do, including embeddings, attention, and feedforward neural networks. We’ll also review the differences between encoder-only, decoder-only, and encoder-decoder models, and the types of LLMs that fall into each category.
Last but not least, we’re going to apply what we’ve learned with Python. We’ll be using Hugging Face’s Transformers library and their Model Hub to demo six practical NLP applications, including Sentiment Analysis, Named Entity Recognition, Zero-Shot Classification, Text Summarization, Text Generation, and Document Similarity.
If you're an aspiring or seasoned data scientist looking for a practical overview of both traditional and modern NLP techniques in Python, this is the course for you.
COURSE CONTENTS:
12.5 hours on-demand video
13 homework assignments
4 interactive exercises
2 skills assessments (1 benchmark, 1 final)
COURSE CURRICULUM:
- Welcome to the Course!
- Benchmark Assessment
- Course Introduction
- About This Series
- Course Structure & Outline
- DOWNLOAD: Course Resources
- The Course Assignments
- Setting Expectations
- Section Introduction
- Anaconda Overview
- Installing Anaconda
- Launching Jupyter Notebook
- Conda Environments Overview
- Conda Workflow
- Conda Commands
- DEMO: Create a Conda Environment
- Environments in This Course
- Section Introduction
- Intro to NLP
- History of NLP
- NLP Applications & Techniques
- NLP Libraries in Python
- Key Takeaways
- Section Introduction
- NLP Pipeline
- Text Preprocessing Overview
- ASSIGNMENT: Create a New Environment
- SOLUTION: Create a New Environment
- Text Preprocessing with Pandas
- DEMO: Text Preprocessing Setup
- DEMO: Text Preprocessing with Pandas
- PRO TIP: Create a Function
- ASSIGNMENT: Text Preprocessing with Pandas
- SOLUTION: Text Preprocessing with Pandas
- Text Preprocessing with spaCy
- Tokenization
- Lemmatization
- Stop Words
- Parts of Speech Tagging
- DEMO: Tokens, Lemmas & Stop Words
- PRO TIP: The Apply Method
- DEMO: Parts of Speech Tagging
- DEMO: Create an NLP Pipeline
- ASSIGNMENT: Text Preprocessing with spaCy
- SOLUTION: Text Preprocessing with spaCy
- Vectorization
- Count Vectorizer in Python
- DEMO: Count Vectorizer
- DEMO: Count Vectorizer Parameters
- PRO TIP: Exploratory Data Analysis
- ASSIGNMENT: Count Vectorizer
- SOLUTION: Count Vectorizer
- TF-IDF
- TF-IDF Vectorizer in Python
- DEMO: TF-IDF Vectorizer
- ASSIGNMENT: TF-IDF Vectorizer
- SOLUTION: TF-IDF Vectorizer
- Key Takeaways
- Section Introduction
- What is Machine Learning?
- Common ML Algorithms for NLP
- Traditional NLP Overview
- Traditional vs Modern NLP
- DEMO: Create a New Environment
- Sentiment Analysis
- Sentiment Analysis in Python
- DEMO: Sentiment Analysis in Python
- ASSIGNMENT: Sentiment Analysis
- SOLUTION: Sentiment Analysis
- Text Classification Basics
- Text Classification Algorithms
- Naive Bayes
- Naive Bayes in Python
- DEMO: Naive Bayes Setup
- DEMO: Naive Bayes Workflow
- DEMO: Naive Bayes Prediction
- PRO TIP: Compare ML Models
- Text Classification Next Steps
- ASSIGNMENT: Text Classification
- SOLUTION: Text Classification
- Topic Modeling Basics
- Topic Modeling Algorithms
- Non-Negative Matrix Factorization (NMF)
- NMF in Python
- DEMO: Fit an NMF Model
- PRO TIP: Display Topics Function
- DEMO: Tune an NMF Model
- Topic Modeling Next Steps
- PRO TIP: Combine ML Algorithms
- ASSIGNMENT: Topic Modeling
- SOLUTION: Topic Modeling
- Key Takeaways
- Section Introduction
- Modern NLP Overview
- Intro to Neural Networks
- Logistic Regression Refresher
- Logistic Regression: Visually Explained
- Neural Networks: Visually Explained
- Neural Network Summary
- EXERCISE: Neural Network Components
- SOLUTION: Neural Network Components
- Neural Networks in Python
- DEMO: Neural Networks in Python
- DEMO: Neural Network Matrices
- PRO TIP: Neural Network Notation & Matrices
- How a Neural Network is Trained
- Neural Network Training: Visually Explained
- EXERCISE: Neural Network Training
- SOLUTION: Neural Network Training
- Intro to Deep Learning
- Deep Learning Architectures
- Deep Learning in Practice
- Pretrained Deep Learning Models
- EXERCISE: Deep Learning Concepts
- SOLUTION: Deep Learning Concepts
- Key Takeaways
- Section Introduction
- Modern NLP Recap
- Transformers & LLMs Overview
- Transformer Architecture
- Transformer Architecture | Embeddings
- Transformer Architecture | Attention
- Transformer Architecture | Feedforward Neural Network
- Transformers Summary
- Breaking Down the Transformer Diagram
- Encoders & Decoders
- Large Language Models (LLMs)
- EXERCISE: Transformers & LLMs Concepts
- SOLUTION: Transformers & LLMs Concepts
- Key Takeaways
- Section Introduction
- Hugging Face Overview
- DEMO: Create a New Environment
- Sentiment Analysis with LLMs
- DEMO: Sentiment Analysis with LLMs
- PRO TIP: Speed Up Transformers Code
- ASSIGNMENT: Sentiment Analysis with LLMs
- SOLUTION: Sentiment Analysis with LLMs
- Named Entity Recognition
- DEMO: Named Entity Recognition
- ASSIGNMENT: Named Entity Recognition
- SOLUTION: Named Entity Recognition
- Zero-Shot Classification
- DEMO: Zero-Shot Classification
- ASSIGNMENT: Zero-Shot Classification
- SOLUTION: Zero-Shot Classification
- Text Summarization
- DEMO: Text Summarization
- ASSIGNMENT: Text Summarization
- SOLUTION: Text Summarization
- PRO TIP: Text Generation
- Document Embeddings
- Cosine Similarity
- Document Similarity with Embeddings
- DEMO: Feature Extraction & Embeddings
- DEMO: Cosine & Document Similarity
- PRO TIP: Recommender Function
- ASSIGNMENT: Document Similarity
- SOLUTION: Document Similarity
- Key Takeaways
- NLP Review & Flow Chart
- NLP Next Steps
- Final Assessment
- Course Feedback Survey
- Share the Love!
- Next Steps
WHO SHOULD TAKE THIS COURSE?
Aspiring Data Scientists who want a practical overview of natural language processing techniques in Python
Seasoned Data Scientists looking to learn the latest NLP techniques, such as Transformers, LLMs and Hugging Face
WHAT ARE THE COURSE REQUIREMENTS?
- We strongly recommend taking our Data Prep & EDA course first
- Jupyter Notebooks (free download, we'll walk through the install)
- Familiarity with base Python and Pandas is recommended, but not required
Start learning for FREE, no credit card required!
Every subscription includes access to the following course materials
- Interactive Project files
- Downloadable e-books
- Graded quizzes and assessments
- 1-on-1 Expert support
- 100% satisfaction guarantee
- Verified credentials & accredited badges
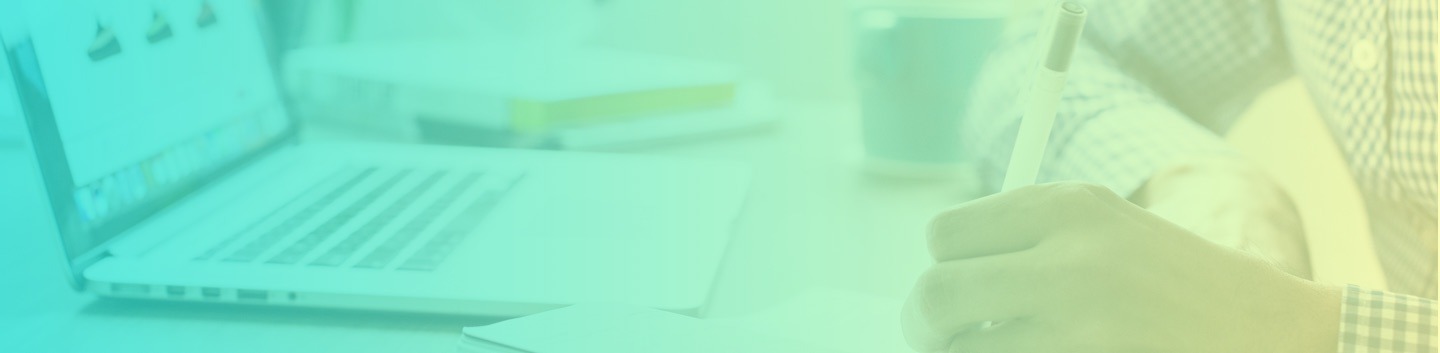
Ready to become a
data rockstar?
Start learning for free, no credit card required!